In the world of software development, writing clean, maintainable, and scalable code is a priority. One of the most effective ways to achieve this in .NET C# is by following the SOLID principles. These principles provide a strong foundation for creating robust software that can adapt to changing requirements and minimize technical debt. In this blog, we’ll dive deep into each of the SOLID principles and will see some examples of each principles.
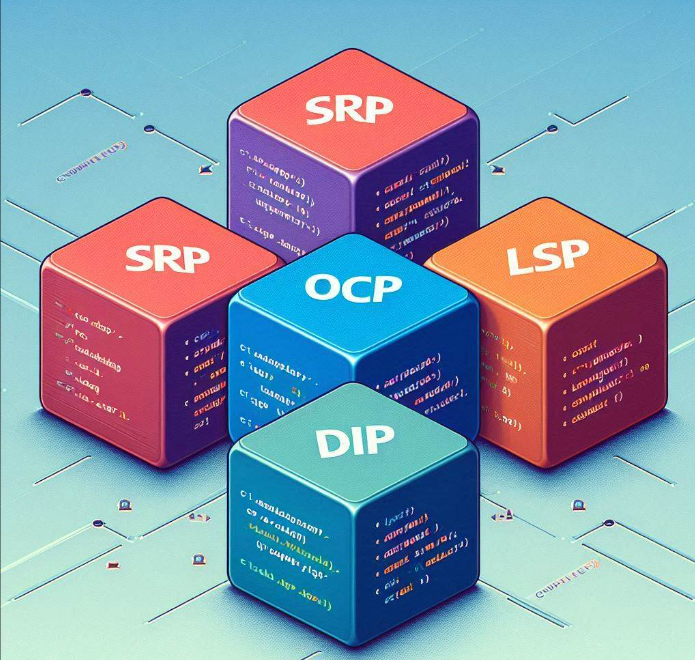
What Are SOLID Principles?
SOLID is an acronym for five key design principles that promote good software design:
- S: Single Responsibility Principle (SRP)
- O: Open/Closed Principle (OCP)
- L: Liskov Substitution Principle (LSP)
- I: Interface Segregation Principle (ISP)
- D: Dependency Inversion Principle (DIP)
These principles are not just theoretical; they are practical guidelines that can help you write code that’s easy to understand, test, and maintain. Let’s explore each principle with examples in C#.
1. Single Responsibility Principle (SRP)
Definition: A class should have only one reason to change, meaning it should have only one responsibility.
Example: Imagine you have a class that handles both user authentication and logging. This violates SRP because it has more than one responsibility. Instead, you should separate these concerns into different classes.
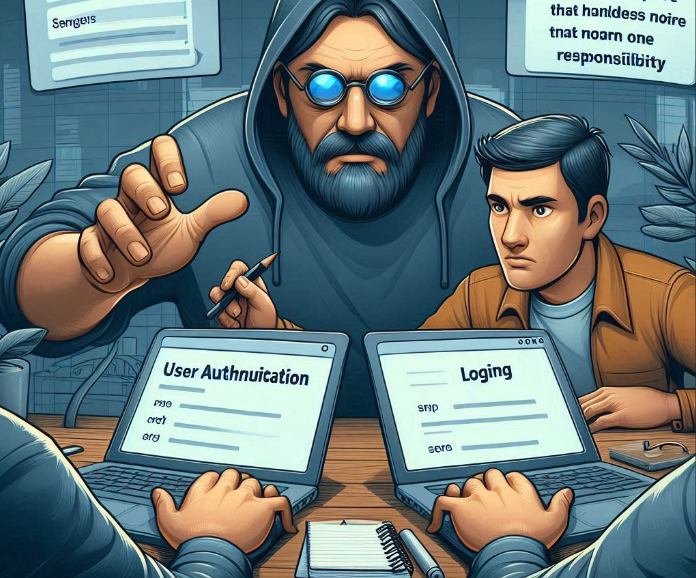
public class AuthenticationService
{
public void AuthenticateUser(string username, string password)
{
// Authentication logic
}
}
public class Logger
{
public void Log(string message)
{
// Logging logic
}
}
2. Open/Closed Principle (OCP)
Definition: Software entities should be open for extension but closed for modification.
Example: Suppose you have a class that calculates the area of different shapes. Instead of modifying the class every time you add a new shape, you can extend it using inheritance or interfaces.
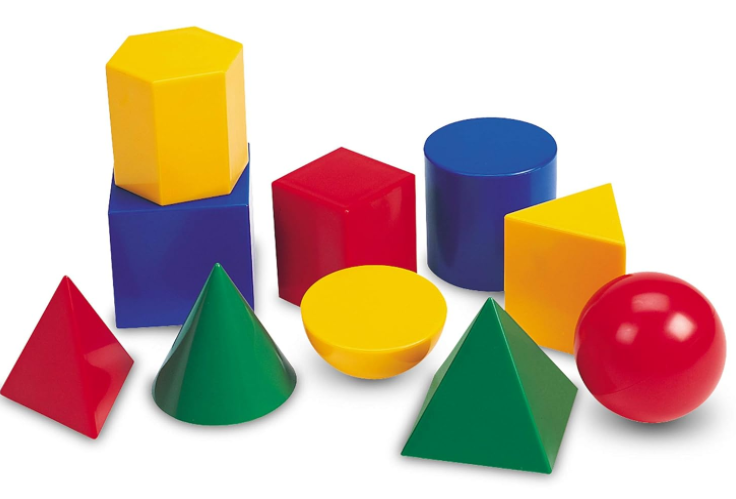
public abstract class Shape
{
public abstract double CalculateArea();
}
public class Circle : Shape
{
public double Radius { get; set; }
public override double CalculateArea()
{
return Math.PI * Radius * Radius;
}
}
public class Rectangle : Shape
{
public double Width { get; set; }
public double Height { get; set; }
public override double CalculateArea()
{
return Width * Height;
}
}
3. Liskov Substitution Principle (LSP)
Definition: Objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program.
Example: If you have a base class Bird
and a derived class Penguin
, you should be able to replace instances of Bird
with Penguin
without breaking the program. However, if Penguin
cannot fly, it might violate LSP if the base class assumes all birds can fly.
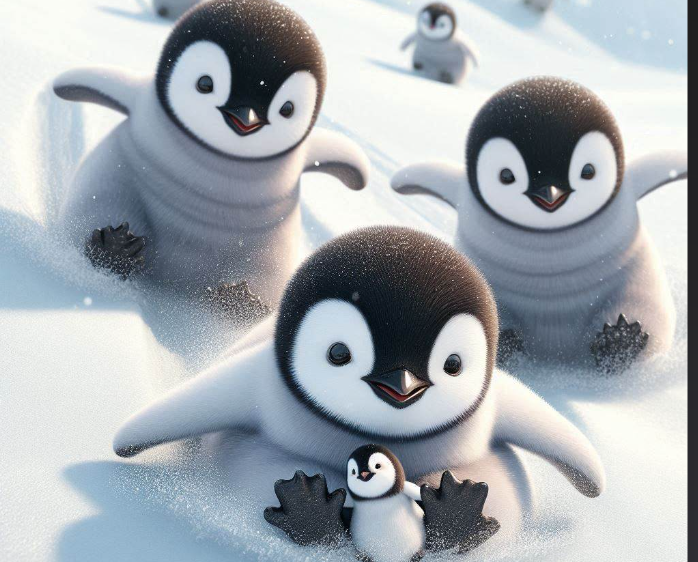
public class Bird
{
public virtual void Fly()
{
// Flying logic
}
}
public class Penguin : Bird
{
public override void Fly()
{
throw new NotImplementedException("Penguins can't fly");
}
}
To adhere to LSP, you can refactor the design:
public abstract class Bird
{
// Bird-specific behavior
}
public class FlyingBird : Bird
{
public void Fly()
{
// Flying logic
}
}
public class Penguin : Bird
{
// Penguin-specific behavior
}
4. Interface Segregation Principle (ISP)
Definition: Clients should not be forced to implement interfaces they don’t use.
Example: If you have an interface IEmployee
with methods for both full-time and contract employees, contract employees might not need all the methods. This violates ISP.
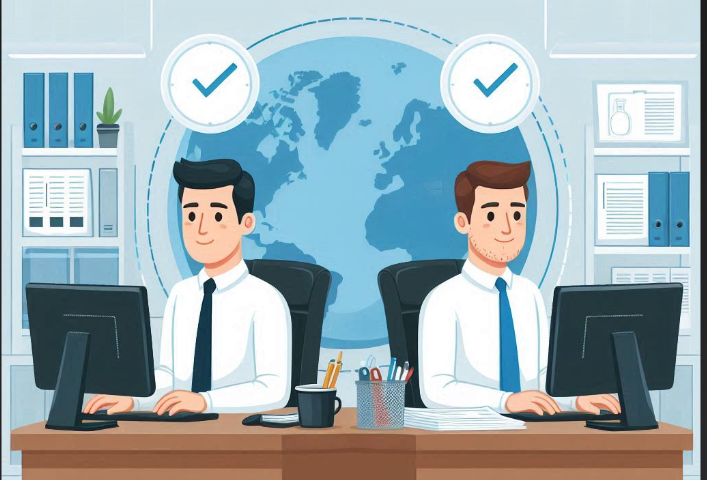
public interface IEmployee
{
void GetSalary();
void GetContractDuration();
}
Instead, segregate the interface:
public interface IFullTimeEmployee
{
void GetSalary();
}
public interface IContractEmployee
{
void GetContractDuration();
}
5. Dependency Inversion Principle (DIP)
Definition: High-level modules should not depend on low-level modules. Both should depend on abstractions.
Example: If a class directly depends on a concrete implementation of another class, it violates DIP. Instead, depend on abstractions (e.g., interfaces).
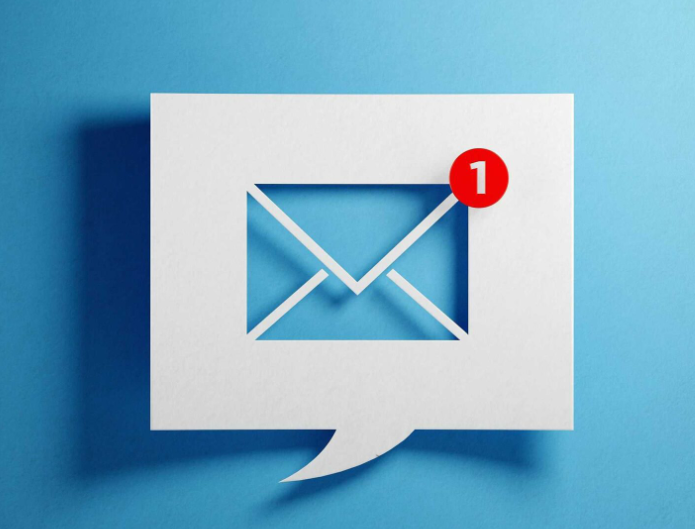
public class EmailService
{
public void SendEmail()
{
// Send email
}
}
public class Notification
{
private EmailService _emailService = new EmailService();
public void Notify()
{
_emailService.SendEmail();
}
}
To adhere to DIP:
public interface IMessageService
{
void SendMessage();
}
public class EmailService : IMessageService
{
public void SendMessage()
{
// Send email
}
}
public class Notification
{
private IMessageService _messageService;
public Notification(IMessageService messageService)
{
_messageService = messageService;
}
public void Notify()
{
_messageService.SendMessage();
}
}
Conclusion
By following SOLID principles in .NET C#, you can create code that is easier to maintain, extend, and scale. These principles help in reducing code complexity, making your applications more robust and adaptable to change. Start implementing SOLID principles in your projects today to experience the benefits of cleaner and more reliable code.
Thanks for stopping by! Your visit means a lot. Please Follow me😊 Stay tuned for more content. Exciting stuff coming your way soon! 🚀 #StayTuned. Also, visit on RioTech.
Comments
Post a Comment